ESign Online API
API Base URL: https://dev.esign.webnerserver.com/api/API Site URL: https://dev.esign.webnerserver.com/
Terms and Conditions: https://winsurtech.com/terms-and-conditions
SLA: https://winsurtech.com/winsurtech-rest-apis-service-level-agreement
Introduction
ESign Online API makes it very easy to accomplish the following:
- Login
- Create Templates
- Create Documents
- Template List
- Prepare Documents
- Send Documents
- Track Documents
- Clients List
- Placeholder List
- Add Client
- Edit Client
- Refresh Token
- Logout
Requirements
- Register on https://esign.winsurtech.com/ before using the ESign Online API
- If you are using POSTMAN to test the APIs then make sure you have POSTMAN version 5.3.0 or above, because below this version you won’t find the “Bearer token” in Authorization.
How to download postman collection
- Login to the site with your credentials - https://dev.esign.webnerserver.com/user-login/
- Go to subscriptions tab - https://dev.esign.webnerserver.com/subscriptions/
- On this page, click on the Download Postman Collection from the link as shown in the screenshot below:
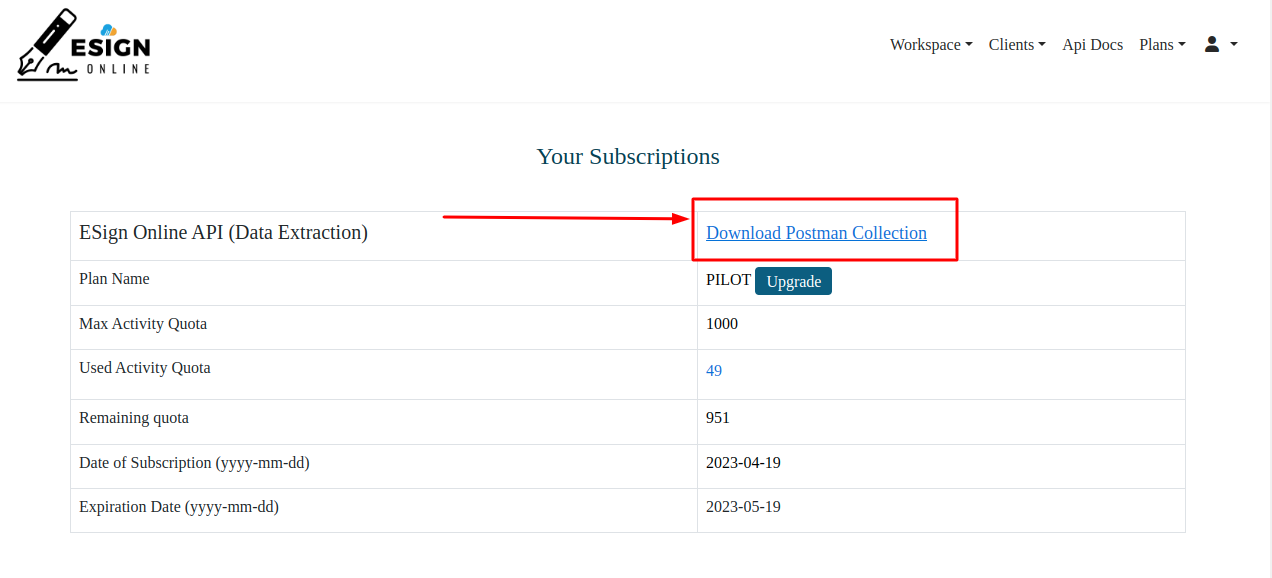
- Import this collection to Postman. The secret key is automatically included in the postman collection.
Setup Enviroment Variable
Base URL
Endpoint: https://dev.esign.webnerserver.com
Postman Screenshot

API List
Login
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/login
Description
This endpoint allows you to login to ESign Online with your mailbox credentials. You need to provide email , password. By providing these details, an access_token and Refresh token is generated which is used to pull files and their content from the mailbox.
Input Parameters
Key (Body Parameter) |
Value |
|
Example: XXXXX@email.com |
password |
Example: XXXXXXXX |
In the Body Parameters (all required):
- email: registered email account with ESign Online
- password: password set by user at the time of registration
Success Sample JSON:
{ "token_type": "Bearer", "expires_in": 1799, "access_token": "eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6ImE1MTQ0NGI5NzhjNmIzNDc4OTY2NTVkMzI5YjVmZWM3MzJlNTQ3OTk3NzVmYWE1YTNhNzMxN", "refresh_token": "def50200f0d26f531b621bb020d1efbc0e4b39730e3e26934acd22220a2d522ecdc6e941b118d8e973f8d5f640b9f30e774086fd9928db7737d2faa428bbe534fd34a8955" }
Failure Sample JSON (in case of invalid credentials)
{ "success": false, "error": "Unauthorised" }
Failure Sample JSON (in case of blank credentials)
{ "success": false, "error": { "email": [ "This field is required" ], "password": [ "This field is required" ] } }
Postman Screenshot
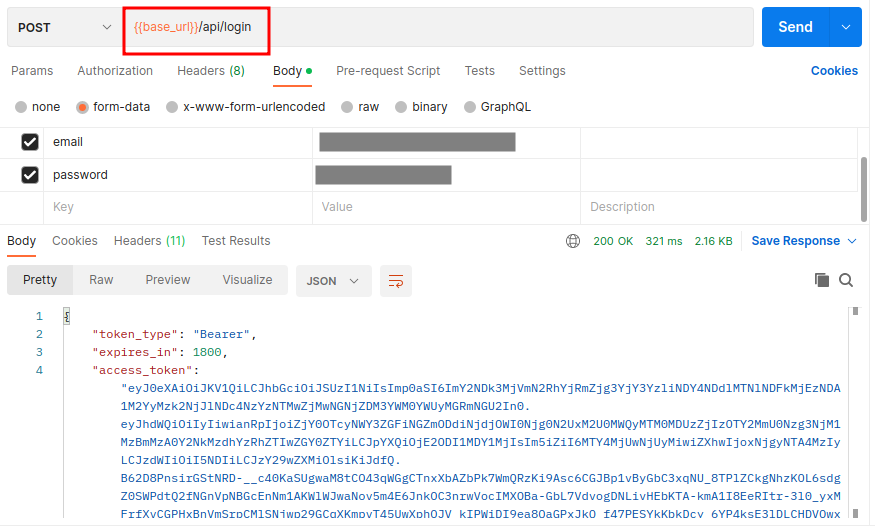
Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/login', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('email' => 'your.email@domain.com','password' => 'your@password'), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Create Template
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/create-template
Description
The Create Template endpoint is used to upload a file that becomes a template and it can be used any number of times to prepare and send documents. You can upload files that have .docx format. You can also replace an existing template document using this endpoint by passing an existing unique_template_name. Once a template is uploaded, it will give you a success response and return a unique template code that can be used to call other endpoints.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
template_file |
Example: Use .docx or .pdf format files |
unique_template_name |
Example: Any Unique template name |
replace_existing |
Example: pass the yes or no value in it |
Success Sample JSON
{ "http_code": 200, "total_time": "8.90345", "status": true, "message": "output is generated", "output_format": "json", "output": { "unique_template_name": "WinsurTech Test 101", "unique_template_code": "NGWXED" } }
Failure Sample JSON (in case template name is not unique and replace_existing is blank or false)
{ "success": false, "error": "A Template with this name already exists, please try with a different one." }
Failure Sample JSON (in case of insufficient inputs)
{ "success": false, "error": { "template_file": [ "This field is required" ], "unique_template_name": [ "This field is required" ] } }
Failure Sample JSON (in case of invalid template file extension)
{ "success": false, "error": "Only .docx, .pptx,.pdf formats are allowed" }
Postman Screenshot
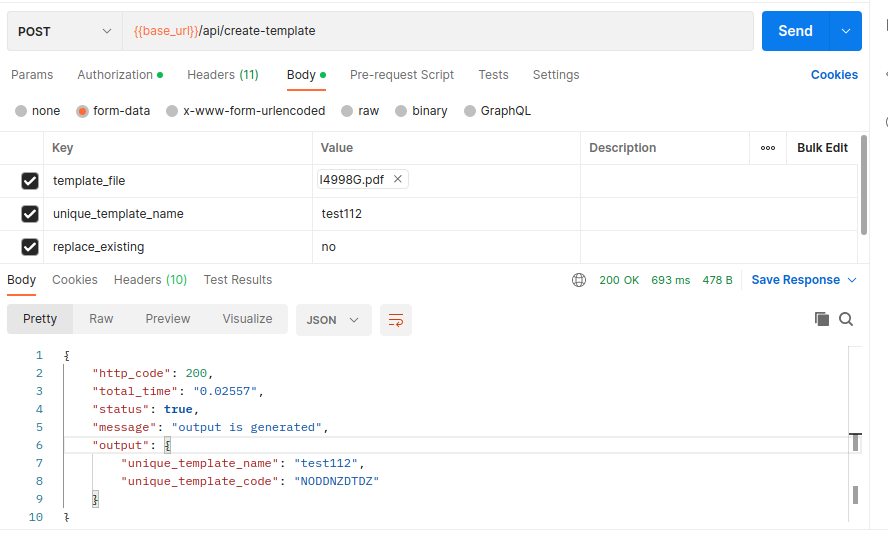
Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/create-template', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('template_file'=> new CURLFILE('/your_folder/docs/creek Hollow 2019 document.docx'),'unique_template_name' => 'WinsurTech',), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6IjQyZjViNTMxOTUxODEx', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Create Document
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/create-document
Description
This endpoint is used to create documents from the templates created in your account. This endpoint takes unique_template_code as input and returns back a unique_document_code which is the id for the document just prepared and it is the required parameter to call some other endpoints.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
unique_template_code |
Example: QLKT3K |
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/create-document', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => 'template_code=6M7OYZ', CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6IjQyZjViNTMxOTUxO', 'Accept: application/json', 'Content-Type: application/x-www-form-urlencoded' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Template List
Request Method:
GET
Endpoint:
https://dev.esign.webnerserver.com/api/template-list
Description
This endpoint gives you the information about your templates like id, template-code, unique-template-name, template-file-name and created timestamp in json format.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/template-list', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6IjQyZjViNTMxOTUxODExY2M', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Prepare Document
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/prepare-document
Description
This endpoint is used to prepare documents (after creating them) before you send them to your prospective users. Before preparing a document you have to create the document using Create Document endpoint. This endpoint takes unique_document_code and variable replacement json content which will set your desired values in the document to prepare the template with the variable data specific to your customer.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
unique_document_code *input_json *
Sample JSON |
|
---|
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/prepare-document', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS =>'{ "unique_document_code": "S5987S", "input_json": { "JsonData": [ { "fieldtype": "table", "fieldName": "table_birth_certificate", "values": [ { "date": "22 Jan, 2021", "location": "Alaska", "address": "142 Creek Hollow Lane Middleburg,Florida 32068", "description": "When an unknown printer took a galley of type" } ] }, { "sendTo": [ "ankush.garg@webners.com" ] } ] } }', CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6IjQw..........HYfaEWKztRjT6k', 'Accept: application/json', 'Content-Type: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Send Document
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/send-document
Description
This endpoint is used to send prepared documents to your prospective users. This endpoint takes unique_document_code, Due_date and document as input and returns a summary of the status and email ids of recipients.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
unique_document_code |
Example: B7557K |
due_date |
Example: yyyy-mm-dd |
document |
Example: Any Message to send the document |
Postman Screenshot
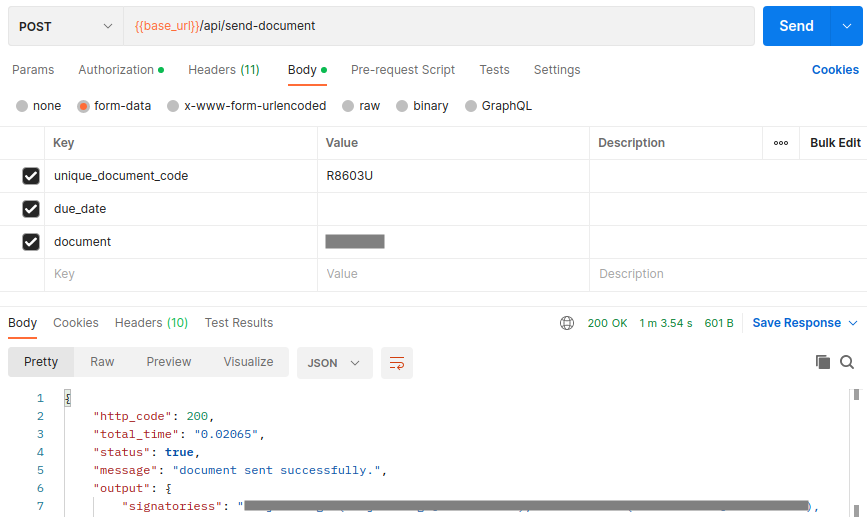
Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/send-document', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('unique_document_code' => 'K1658Y','due_date' => '2021-12-25','document' => 'Sample message'), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Track Document
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/track-document
Description
This endpoint is used to track your document status. It takes unique_document_code as input and returns the status of the document and other information like unique_document_name, unique_document_code, document status
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
unique_document_code |
Example: B7557K |
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/track-document', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('unique_document_code' => 'K1658Y'), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0aSI6IjQyZjViNTMxOTUxODExY', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Clients List
Request Method:
GET
Endpoint:
https://dev.esign.webnerserver.com/api/clients-list
Description
This endpoint gives you the information about your clients like id, first_name, last_name, company_name, email, title, street_address_1, street_address_2, city, country, zipcode, mobile_number, landline_number, website_url, fax_number, users_id, company_id, signatures, status etc in json format.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/clients-list', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'GET', CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Placeholder List
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/placeholder-list
Description
This endpoint gives the information about placeholders of all field types like table, bullet list, strings. It takes the unique_template_code as a input parameter and return all the placeholders of relative template.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
unique_template_code |
Example: O52VKZ |
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/placeholder-list', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('unique_template_code' => 'O52VKZ'), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Add Client
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/add-client
Description
This endpoint is used to create a new client by adding the details of the client like first_name, last_name, email_id and another optional fields company_name, company_url, city, country, zip code etc as input parameters. In this endpoint, email_id, first_name and last_name input parameters are mandatory.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
first_name |
Example: XXXXX |
last_name |
Example: XXXXX |
|
Example: XXXXXX@email.com |
company_name |
Example: XXXXX |
company_url |
Example: example.com |
street_address_1 |
Example: :street address 1 |
street_address_2 |
Example: street address 2 |
city |
Example: Mohali |
state |
Example: Punjab |
country |
Example: India |
zipcode |
Example: 160071 |
mobile_number |
Example: +9180XXXXXX00 |
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/add-client', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('first_name' => 'XXXXX','last_name' => 'XXXXX','email' => 'XXXXX','company_name'=>'company'), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Edit Client
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/edit-client
Description
This endpoint is used to edit the existing client details like email_id, first_name, last_name and another optional fields company_name, company_url, city, country, zip code etc as input parameters. In this endpoint email input parameter is mandatory.
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Input Parameters
Key (Params Parameter) |
Value |
|
Example: XXXXXX@email.com(Existing client email) |
new_email |
Example: : ZZZZZZ@email.com(New email that needs to update) |
first_name |
Example: XXXXX |
last_name |
Example: XXXXX |
company_name |
Example: XXXXX |
company_url |
Example: example.com |
street_address_1 |
Example: street address 1 |
street_address_2 |
Example: street address 2 |
city |
Example: Amritsar |
state |
Example: Punjab |
country |
Example: India |
zipcode |
Example: 143001 |
mobile_number |
Example: +9180XXXXXX00 |
Postman Screenshot
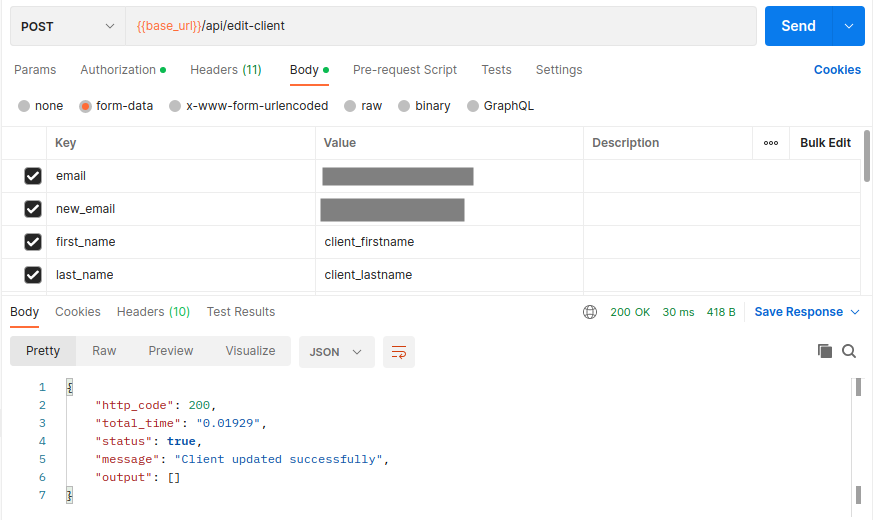
Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/edit-client', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('email' => ' XXXXXX@email.com','new_email' => 'ZZZZZZ@email.com','first_name' => 'XXXXX','last_name'=>'XXXXXX','company_name'=>'XXXXXX','company_url'=>'example.com'), CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Refresh Token
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/refresh
Description
The Refresh Token endpoint is used to get a new Auth access token while passing a refresh token which was received in Login API/Refresh Token API so that currently login user stay active in App.
Input Parameters
Key (Params Parameter) |
Value |
refreshToken |
Example: Added the refresh token returned by the login API |
Success Sample JSON:
{ "token_type": "Bearer", "expires_in": 2592000, "access_token": "new access token key", "refresh_token": "refresh token key" }
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/refresh', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_POSTFIELDS => array('refreshToken' => 'def50200b53ff2043a442c70e58dd4216e719a1b76e1b4dc3cec3b80903ef5f'), CURLOPT_HTTPHEADER => array( 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;
Logout
Request Method:
POST
Endpoint:
https://dev.esign.webnerserver.com/api/logout
Description
This endpoint is used to Logout from API
Headers
Authorization |
Value |
Bearer token |
Example: Added the access token returned by the login API |
Accept: application/json |
Example: to receive data in json format |
Failure JSON (in case of invalid credentials):
{ "http_code": 401, "total_time": "0.16094", "status": true, "message": "You are not authorized to complete this request, please login and try again!", "output_format": "json", "output": [] }
Postman Screenshot

Sample code in PHP
$curl = curl_init(); curl_setopt_array($curl, array( CURLOPT_URL => 'https://esign.winsurtech.com/api/logout', CURLOPT_RETURNTRANSFER => true, CURLOPT_ENCODING => '', CURLOPT_MAXREDIRS => 10, CURLOPT_TIMEOUT => 0, CURLOPT_FOLLOWLOCATION => true, CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1, CURLOPT_CUSTOMREQUEST => 'POST', CURLOPT_HTTPHEADER => array( 'Authorization: Bearer eyJ0eXAiOiJKV1QiLCJhbGciOiJSUzI1NiIsImp0a', 'Accept: application/json' ), )); $response = curl_exec($curl); curl_close($curl); echo $response;